"Breakaway Bowling" Dev Diary
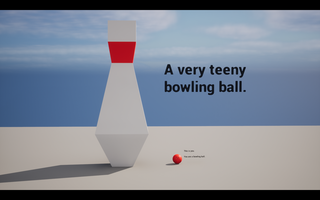
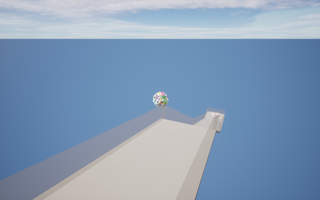
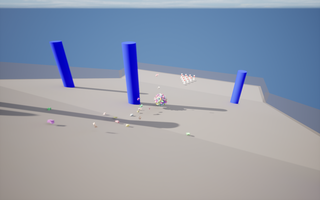
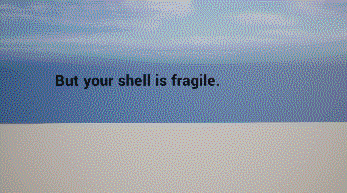
This year's Obsidian Game Jam ran for 26 hours and had the theme of "Teeny". I set out on a solo effort to make a game, and ended up making "Breakaway Bowling," a game where you're a teeny bowling ball wrapped in a shell that breaks apart with every attempt to knock down the pins.
Conception
Given the theme of "Teeny," I immediately wanted to make a game where you got smaller and smaller as you played. Game progression is more likely to be centered on the player avatar getting bigger and more powerful, and I liked the idea of exploring the reverse of that. When thinking of classic "get bigger"-style games, the one that first came to mind was Katamari, where a ball collects objects it rolls over and gets larger and larger – I liked the idea of reversing that and having pieces of a large ball break off, causing it to get smaller and smaller the more it rolled. Making it a bowling ball turned that mechanic into a neat little difficulty curve, since the smaller the ball gets, the harder it becomes to knock stuff down with it.
Given the time constraint of the game jam, the scope would have to be pretty simple. For a minimum viable result, I'd need to get the "pieces breaking off of a ball" mechanic working, have a way to aim and launch the ball, a system for recognizing when pins were knocked down, and at least one level ("lane") to showcase it. Ideally, I'd be able to get multiple lanes and a menu system to switch between them. I'd be making the game in Unreal Engine 5.
The Ball
The basic idea for the bowling ball was to have a small core surrounded by a bunch of individual static mesh components, which would be attached to the core but have the ability to "break off" and become independent physics bodies.
First up, I needed a way to place the individual pieces in a spherical shape. I knew right away that the number of pieces I wanted was going to make manually placing each one unfeasible, so I looked for a programmatic solution. Luckily, smarter people than I have already solved the problem of getting an arbitrary number of points on the surface of a sphere, so I implemented a Fibonacci sphere algorithm to dynamically place the static mesh components (thank you, Stack Overflow). With that solved, it was pretty simple to get the ball rolling by enabling physics on the core and putting it on a slope:
The next step was being able to identify when the ball hit an object. I struggled with this for a bit, since I wanted each individual piece to be able to register its own hit events independently of the others. I tried in vain for a while to get OnComponentHit events working, and I'm still not sure where I was going wrong. I tried a bunch of permutations of collision and physics options for all of the meshes involved, but they didn't give me the results I wanted (and often made things worse).
Ultimately, I fell back to using the hit events for the bowling ball actor as a whole instead of the events for individual components. The ball would properly register hits when any of its static mesh components impacted something, but that left me without a way to immediately determine which piece to break off (in hindsight I think this is wrong; I think the individual component that impacted something is passed into the actor's hit event, but I didn't know this at the time). I ended up running a check with each hit to find the piece (or pieces) closest to the hit location in world space, and using that to select which piece(s) to break off.
In order to "break off" a piece, I set it up to spawn in a new, independent static mesh actor with the same properties as the soon-to-be-broken static mesh component, give the new actor a little velocity to try to push it away from the ball, and destroy the original component. The resulting illusion was pretty seamless.
Since physics collisions can happen dozens of times per second, I added a brief (~1 second) cooldown between pieces breaking. I also wanted to only break off pieces when the impact was, well, impactful enough (as well as break off more pieces for extra big impacts). I expected Unreal's OnHit events to pass along some info about the "size" of the impact, and was disappointed to find that that wasn't the case. I ended up trying multiple methods here that didn't work, and ended up with a solution that I'm not totally happy with, but it was good enough that it didn't seem smart to spend more time struggling with it. I tried using the angle between the ball's velocity and the impact normal vector to tell if the impact was opposing the direction of motion (e.g. hitting a wall rather than bouncing along the ground) but that didn't work reliably; it looks like the ball's velocity change when impacting something happens before the hit event is processed, meaning by the time I was querying the velocity, the ball was already in the process of bouncing back the way it came. I tried storing the ball's velocity for each previous tick and watching for a significant change from one tick to the next, and I feel like that method had promise but I wasn't able to get it working. Ultimately I needed to move on, so I went with a simple method of "if the ball is going fast when it hits something, break off a piece". The faster the ball is moving when it hits something, the more pieces break off. The result is that a ball rolling quickly but smoothly down a hill will continuously hemorrhage pieces, which isn't what I had wanted – I had imagined pieces breaking off only when hitting a wall or an obstacle – but I accepted it.
The resulting blueprint script is a tangled mess of dead branches for abandoned methods and attempts at debugging, but I was long past trying to make it pretty.
The Pieces
In a different world, the pieces making up the ball's shell would have been recognizable objects in the vein of Katamari, but 3D modeling is not yet one of the tools I have in my belt.
I suppose I could have found some nice low-poly models for free somewhere, but that didn't seem to be in the spirit of the game jam (and also I have a masochistic drive to never use ready-made solutions if it's at all possible for me to do it myself – I will create so much extra work for myself rather than downloading assets and it's probably not a great habit). I also could have just kept using primitive shapes like cubes and cylinders, but I wanted a lot more variety than that in the various pieces. So instead, I turned to my old friend, procedural mesh generation.
I've worked with Unreal's Procedural Mesh Component a bit in the past, so I had a good idea of how to tackle it. The idea was to set up a few basic mesh shapes and allow the locations of each vertex to have random variation so each piece would come out as its own unique shape. I wanted the shapes to have enough flexibility to allow for lots of variations, but without getting so complex that the logic for constructing the procedural mesh broke my brain and took the entire 24 hours.
I sketched out the basic meshes of a few shapes that I'd use for the ball's pieces, plus one that I'd eventually use to make the pins. I ended up moving on after implementing only one of these, and I'm very glad that I did – I eventually found that you couldn't even see enough details in the pieces while the ball's moving and chunks are flying off to notice the variations, and I had greatly overemphasized the need for variety. The basic framework of two rectangular prisms stacked on top of each other, once the vertices all got their little perturbations, was enough to produce all of the variation I needed.
Rather than actually use the procedurally-generated meshes at runtime, I made a couple dozen variants and saved them off as static meshes. This avoided the performance cost of trying to create potentially hundreds of meshes all at once. Now, when the ball was spawned, it picked from a random list of abstract shapes, slapped on a simple dynamic material instance with a random color, and used those for all of the pieces of its shell. The result worked perfectly right away.
... okay, not really. Turns out there were some issues with collision on these meshes – the procedural-mesh-to-static-mesh pipeline can generate complex collision, but not simple collision, which meant it caused trouble for physics simulations. I ended up having to go back through all of the static meshes I had generated and generate new simple collision for them manually. It was a far cry from the "magically get a hundred unique meshes" process I had imagined, and another reason I'm glad I didn't spend more time trying to get more mesh variety.
At this point, I had the basic mechanics of the ball working (albeit with some material issues on the broken pieces) and I was feeling good about being able to end up with a finished product by the end of the jam.
The Pins
The bowling pins ended up being pretty simple. I used the same procedural-to-static mesh process as above to make a custom pin mesh, and set up a blueprint that waited for its "up" vector to be pointed sufficiently away from vertical, at which point it would consider itself tipped over. Once all of the pins were tipped, the player would win.
Later, I'd go back and re-make the pin mesh to allow for the iconic red stripe along the "neck", which I was happy with.
The Launcher
The launch mechanism also ended up being relatively simple to set up. It used a simple arrow to show where it was pointed, and could change pitch, yaw, and launch force. With the press of a button, it would enable physics on the bowling ball and set it moving in the specified direction.
The Camera and Player Controller
Getting the camera working and processing player input correctly ended up being a huge pain. This is mostly uncharted territory for me – when I'm tinkering in Unreal, I'm usually content to just set up neat little blueprints and maps for myself, and use Unreal's built-in player movement stuff. Here, I needed to have a different behavior for when the player was aiming the launcher and when the ball was rolling, and it was a ton of trial-and-error trying to figure out how to set up my own player controller, player pawn, and game mode. It's likely the kind of thing I could have solved by spending an hour reading or watching tutorials, but instead I stubbornly tried to figure it out for myself and ultimately spent longer to get it all hacked together.
Eventually, I had a system where the camera would be static when the player was aiming, and once the ball was launched, the camera would find a location behind, above, and a bit to the side of the ball and try to follow it. It ended up being... fine. It worked, but I would have liked it to be better. When aiming, the fixed camera doesn't really let you see enough about the lane, and the perspective can make it difficult to tell how much the arrow is pitched up/down. I'm not terribly happy with it, but considering how much I struggled to get even this working, I was willing to leave well enough alone.
Building Lanes
At this point, I was practically already at the minimum viable result with time to spare, so I set out to make a handful of lanes. I tried exploring a few different kinds of concepts, like a pachinko-style set of obstacles, a big hole in the middle of the lane to avoid, and needing to bounce the ball off of a floating island. I probably would have made a lot more lanes but the process honestly was just kind of a drag. I was stuck using basic primitive shapes and trying to piece them together, which meant that straight lines were easy but curves were nearly impossible. I likely could have done a lot more with some kind of mesh sculpting tools, but all of that is uncharted territory for me, and given the time constraints I didn't really have the luxury to explore it.
The Intro
Over the course of development, I had started to really personify the bowling ball. The "core" of the ball ended up having the classic three finger holes, and I very much appreciated that they made it look like a little cute face. I was having a lot of fun thinking of the ball as "just a lil guy" rather than just a lifeless ball, and I wanted to communicate that to players. Given that I had time left to spare in the game jam, I set out to make a little intro cinematic.
I set up an empty map and used Unreal's level sequencer to do some simple camera movements and visibility toggling of meshes and text. The most complex part was queueing up a bowling ball to spawn in and bounce across the screen to show off the pieces breaking – it took some time to get the timing and positioning just right, but ended up working out.
The result ended up being my favorite part of the whole thing. I think it's really charming and effective and the little bowling ball guy is just so dang cute.
Conclusion
Overall, I'm really happy with what I ended up making. It's super rare for me to actually finish anything I start – I'm perpetually tinkering with stuff until I get bored of it and moving on to the next thing, without ever producing any actual whole product, so it was really satisfying to push myself through to getting an executable file out into the world.
I think the game itself is a neat concept with some potential, although there's plenty of room for improvement. I would have liked to do a lot more tuning with things like the ball's physics properties. Right now, the ball feels a lot floatier than I'd like – it doesn't have that powerful feeling you get when rolling a bowling ball down a smooth lane, instead everything kind of feels like it's happening a bit in slow motion. The current configuration also doesn't give the sense of drastically changing scale that is emblematic of Katamari – the ball is smaller once it's lost a bunch of pieces, but it doesn't feel like you're in a whole different world or anything. There's probably some tuning that could be done there with the size of the pieces that break off – really big pieces in the outer layer, and really small pieces in the inner layer, for example. The camera could also probably carry a lot of weight here; having the camera get closer the smaller the ball gets could make the pins seem much bigger in comparison.
In the end, though, I think it's great for being made in 24 hours. It's got a little bit of charm, and it's still satisfying to me to watch chunks fly off as the ball rips down towards a strike.
Leave a comment
Log in with itch.io to leave a comment.